Welcome to another blog post that delves into the world of prompt engineering.
Drawing from the well known deeplearning.ai course, I already touched upon the foundations of effective prompting—like writing specific instruction and giving the LLM time to think— and now I want to turn our attention to small yet impactful practical applications that serve as catalysts for endless inspiration.
The first aspect to highlight is how prompt development is an iterative process, that involves outlining an idea in a clear and specific way, observing the results and refining the starting idea until the output is satisfying.
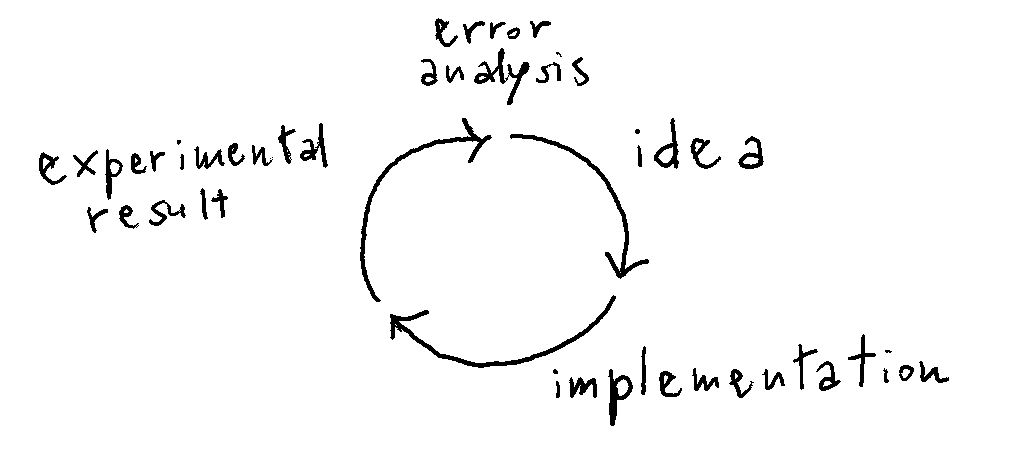
Keeping that to mind, we can outline four fundamental tasks that an LLM can pick up:
The examples described in this post use the setup introduced in this earlier article about fundamentals of effective prompting .
Now let's dive into each task!
Summarising
Let's consider a case study in which we are given customers review and we need to extract from them insightful information for the shipping and delivery department of our e-commerce website.
Just a simple summary of the review is not giving much help in itself, but creating a prompt that outlines the task of the model giving instruction on the aspect to focus on is way more powerful:
n_words = 30
prompt = f"""
Your task is to generate a short summary of a product review from an e-commerce website to give feedback and insightful information to the shipping and delivery department.
Summarize the review delimited by triple backsticks in at most {n_words} words and focusing on any aspect that mentions shipping and delivery of the product.
Review: {review}
"""
This is just an example with a single review, but we can also loop through thousands of them, and find a way to structure the output in a usable manner:
reviews = ["review 1", "review 2", ...] #list of reviews
for i in range(len(reviews)):
prompt = f"""
Your task is to generate a short summary of a product review \
from an e-commerce website to give feedback and insightful \
information to the shipping and delivery department.
Summarize the review delimited by triple backsticks in \
at most {n_words} words and focusing on any aspect that \
mentions shipping and delivery of the product.
Review: {reviews[i]}"""
Inferring
Still considering product reviews, we can ask the model to perform some sort of analysis and extract labels, names and sentiment of a text.
Let's assume we have a very long headphones review:
headphones_review = """
I bought these Soundscape XR headphones as a \
replacement for a Replacement \
of a replacement pair of headphones I had for maybe \
3/4 years until the charging port died. Betron HD800 \
... R.I.P. Then got a pair of KS Engage 2 from here \
on sale. dropped those and now, well, they do a job, \
I sleep with those now.
You can actually feel the pads cancelling the noise \
out on these. As a hypersensitive-hearing woman, you \
seek the best sound quality and noise blocking. \
I haven't been this impressed since the Betron. \
You can hear all of the instruments!
Also, I love music production.
So far it's passing the sound and audio quality \
test of the new headphone playlist on a \
laptop (wired). I don't think it's charged enough \
to use and check the Bluetooth at the moment, so \
will run later tests and add the rest of the review. \
I can't hear my mum in the kitchen and her video XD, \
can just about hear the microwave.
Report back later with the rest.
Day 2 : Went to work.
Bluetooth is good and working fine for the phone. \
Managed to block out the screech of the Tube in the \
tunnels and on the Jubilee line. For once I left the \
train without a pounding headache and anxiety.
It still loud, but not as unbearably loud.
The noise cancelling feature - the button doesn't do \
much actually sounds quieter. The pads and volume is \
good and you can have a peaceful commute :)!
Even recommended to a colleague.
I think in this case Soundamaze Ltd did a very good job. \
Amazing for what they are on a budget for \
noise-cancelling headphones.
"""
Depending on what we want to achieve, there are several applications of the LLM inferring capabilities, just to name a few:
- sentiment labelling (useful for binary classification of negative/positive reviews)
prompt = f"""
What is the sentiment of the following product review,
which is delimited with triple backticks?
Give your answer as a single word, either "positive" \
or "negative".
Review text: '''{headphones_review}'''
"""
- identifying a list of emotions (the prompt below gives as output:
impressed, satisfied, relieved, calm, enthusiastic
)
prompt = f"""
Identify a list of emotions that the writer of the \
following review is expressing. Include no more than \
five items in the list. Format your answer as a list of \
lower-case words separated by commas.
Review text: '''{headphones_review}'''
"""
- identifying a specific emotion, if present in the review (sometimes departments like customers service have value in understanding if the user is really upset for example)
prompt = f"""
Is the writer of the following review expressing anger?\
The review is delimited with triple backticks. \
Give your answer as either yes or no.
Review text: '''{lamp_review}'''
"""
- identifying information like name of the item, company that made it and the best feature in the customer's opinion.
Using the prompt below, the output will look like this:{
"Item": "Soundscape XR headphones",
"Brand": "Soundamaze Ltd",
"Bestfeature": "Noise cancelling pads"
}
prompt = f"""
Identify the following items from the review text:
- Item purchased by reviewer
- Company that made the item
- Most appreciated feature
The review is delimited with triple backticks. \
Format your response as a JSON object with \
"Item", "Brand" and "Bestfeature" as the keys.
If the information isn't present, use "unknown" \
as the value.
Make your response as short as possible.
Review text: '''{headphones_review}'''
"""
All the prompts listed in this section can be combined into a single one, and the fields of application are endless; another example could be a topics inference script to categorize a news article, or to detect if the article is dealing with certain topics.
This simple prompt would lead to a news alert system that would have required more time to be implemented with traditional :
story = """Some story about AI and machines that are able to learn..."""
topic_list = ["AI", "software development", "data science", "technology"]
prompt = f"""
Determine whether each item in the following list of \
topics is a topic in the text below, which
is delimited with triple backticks.
Give your answer as list with 0 or 1 for each topic.\
List of topics: {", ".join(topic_list)}
Text sample: '''{story}'''
"""
Transforming
In addition to translations to other languages, we can create prompts that perform grammar correction, text rephrasing or proof reading, and even convert from one data format to another.
Few examples of prompts performing transformation tasks:
- language detection and translation
user_messages = ["message_1", "message_2",...] #list of users messages
for m in user_messages:
prompt_1 = f"""
Detect the language of the following message: ```{m}```
"""
lang = get_completion(prompt_1)
#the get_completion function is explained in an earlier post
prompt_2 = f"""
Translate the text to English and German: ```{m}```
"""
translation = get_completion(prompt_2)
- changing the tone
prompt = f"""
Translate the following from slang to a business letter:
'Dude, This is your old mate Frank, \
check out this spec on this standing lamp. \
Get out of your hole sometime, let's grab a beer!'
"""
Output:
"""
Dear [Name],
I hope this letter finds you well. I wanted to bring to your attention a specification for a standing lamp that I believe may be of interest to you. Additionally, I would like to extend an invitation to meet up for a drink at your convenience.
Best regards,
[Your Name]
"""
- Data format conversion
data_json = { "resturant employees" :[
{"name":"Shyam", "email":"shyamjaiswal@gmail.com"},
{"name":"Bob", "email":"bob32@gmail.com"},
{"name":"Jai", "email":"jai87@gmail.com"}
]}
prompt = f"""
Translate the following python dictionary from JSON to an HTML \
table with column headers and title: {data_json}
"""
response = get_completion(prompt)
print(response)
Output:
"""
<table>
<caption>Restaurant Employees</caption>
<thead>
<tr>
<th>Name</th>
<th>Email</th>
</tr>
</thead>
<tbody>
<tr>
<td>Shyam</td>
<td>shyamjaiswal@gmail.com</td>
</tr>
<tr>
<td>Bob</td>
<td>bob32@gmail.com</td>
</tr>
<tr>
<td>Jai</td>
<td>jai87@gmail.com</td>
</tr>
</tbody>
</table>
"""
The output can be easily rendered with the IPython
package:
from IPython.display import display, Markdown, Latex, HTML, JSON
display(HTML(response))
Name | |
---|---|
Shyam | shyamjaiswal@gmail.com |
Bob | bob32@gmail.com |
Jai | jai87@gmail.com |
- Proof reading and grammar checking
prompt = f"""
proofread and correct this review. Make it more compelling.
Ensure it follows APA style guide and targets an advanced reader.
Output in markdown format.
Review: ```{text}```
"""
Having the original text saved as text
and the result of the prompt saved as response
, we can visualise the corrections with this simple code:
from IPython.display import display, Markdown
from redlines import Redlines
diff = Redlines(text,response)
display(Markdown(diff.output_markdown))
Expanding
The LLM of choice can also be used as a brainstorming partner once it has been made aware of some information; as an example, we can generate a personalised reply to a reader's comment:
review = "Great article! I really like the technical part about the \
proof-reading prompt, super useful!"
sentiment="positive" #this can be retrieved using one of the prompts above
prompt = f"""
You are mariani.ai website AI Assistant.
Your task is to reply to a valued reader message.
Given the reader message delimited by ```, \
Generate a reply to thank the reader for their message.
If the sentiment is positive or neutral, thank them for \
their comment.
If the sentiment is negative, ask for further feedback \
in order to improve the website content, showing appreciation \
for a constructive criticism.
Make sure to use specific details from the message.
Write in a friendly and confident tone, as if you were \
part of the team creating the article.
Sign the message as `Davide's AI assistant`.
Customer review: ```{review}```
Review sentiment: {sentiment}
"""
response = get_completion(prompt, temperature=0.7)
print(response)
Output:
"""
Dear valued reader,
Thank you so much for taking the time to read our article and
for leaving such a kind comment! We're thrilled to hear that
you found the technical part about the proof-reading prompt useful.
We're constantly striving to provide our readers with informative
and engaging content, so your feedback truly means a lot to us.
If you have any suggestions or topics you'd like to see us cover
in the future, please don't hesitate to let us know.
Thanks again for your support!
Best regards,
Davide's AI assistant
"""
I hope you found this article useful! Don't worry, if you ever leave a comment (I would love that) I will always reply myself!